|
'ITWeb/개발일반'에 해당되는 글 489건
- 2008.02.20 JavaScript DOM
- 2008.02.20 javascript object, class & inheritance, sigletons
- 2008.02.20 내장객체, BOM, DOM, 사용자 정의 객체
- 2008.02.20 objects, collections, linkage, invocations, this, typeof, closure, namespace, inheritance
- 2008.02.20 try-catch-throw, special text
- 2008.02.20 JavaScript Loops
- 2008.02.20 JavaScript Functions
- 2008.02.20 if else statement, switch statement, operators
- 2008.02.20 block, comments, variables
- 2008.02.20 JavaScript Where To
ITWeb/개발일반 2008. 2. 20. 17:34
•Node
–-Retrieving nodes
§document.getElementById(id)
§document.getElementsByName(name)
§node.getElementsByTagName(tagName)
•Node
–Document tree structure
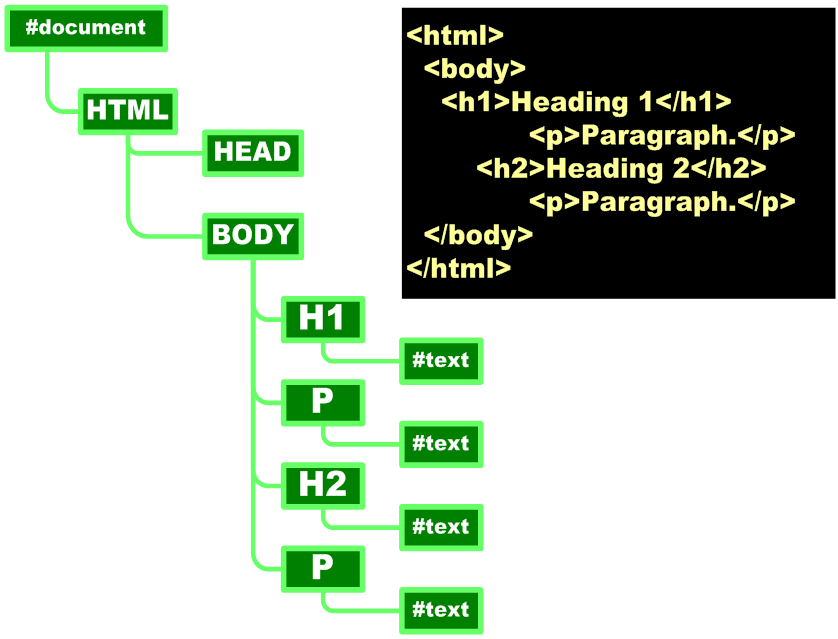
•Node
–-child, sibling, parent 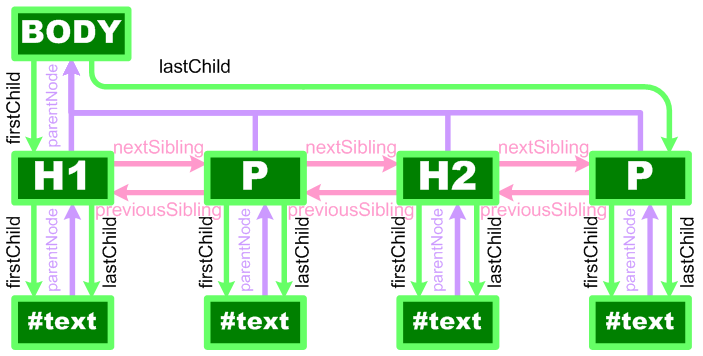
•Walk the DOM
–-Using recursion, follow the firstChild node, and then the nextSibling nodes.
§function walkTheDOM(node, func) {
§ func(node);
§ node = node.firstChild;
§ while (node) {
§ walkTheDOM(node, func);
§ node = node.nextSibling;
§ }
§}
•Style
–-node.className
–-node.style.stylename
–-node.currentStyle.stylename Only IE
–-document.defaultView(). getComputedStyle(node, ""). getPropertyValue(stylename);
§var oDiv = document.getElementById("div1");
§var nHeight = document.defaultView.getComputedStyle(oDiv, null).getPropertyValue("height");
Style names
CSS
•background-color
•border-radius
•font-size
•list-style-type
•word-spacing
•z-index
JavaScript
•backgroundColor
•borderRadius
•fontSize
•listStyleType
•wordSpacing
•zIndex
•Making elements
–-document.createElement(tagName)
§var hr = document.createElement("hr");
§document.body.appendChild(hr);
–-document.createTextNode(text)
§var txt = document.createTextNode("Hello!");
§document.body.appendChild(txt);
–-node.cloneNode(deep);
§cloneNode(true) clones the whole subtree rooted at the node
§cloneNode(false) only the node itself (and any attributes if it is an element) is cloned
–curr_node = root.firstChild;
–var new_node = curr_node.cloneNode(true);
–root.appendChild(new_node);
•Linking elements
–-node.appendChild(new)
–-node.insertBefore(new, sibling)
–-node.replaceChild(new, old)
–-old.parentNode.replaceChild(new, old)
•Removing elements
–-node.removeChild(old)
–-old.parentNode.removeChild(old)
•innerHTML
–-The W3C standard does not provide access to the HTML parser.
–-All A browsers implement Microsoft's innerHTML property.
ITWeb/개발일반 2008. 2. 20. 17:08
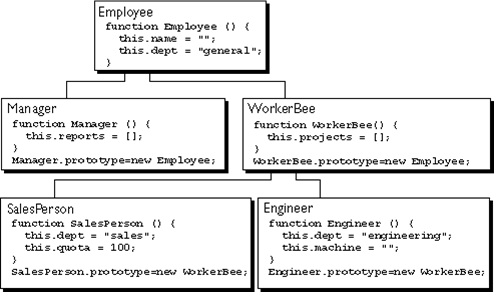
•Class & Inheritance
–-Prototypal Inheritance
var oldObject = {
firstMethod: function () { alert("first"); },
secondMethod: function () { alert("second"); }
};
var newObject = new Object(oldObject);
newObject.thirdMethod = function () { alert("third"); };
var myDoppelganger = new Object(newObject);
myDoppelganger.firstMethod(); // or
myDoppelganer[“firstMethod”](); |
var obj1 = function () {
this.title = "obj1";
}
var obj2 = function () {}
obj2.prototype = new obj1;
var obj2Class = new obj2();
alert(obj2Class.title);
obj1 = {
title:"obj1_"
}
obj2 = new Object(obj1);
alert(obj2.title); |
–-Method apply (Member variable)
§Function.apply(thisArg[, argArray])
–function Car(make, model, year) {
– this.make = make;
– this.model = model;
– this.year = year;
–}
–
–function RentalCar(carNo, make, model, year) {
– this.carNo = carNo;
– Car.apply(this, new Array(make, model, year));
–}
–
–myCar = new RentalCar(2134,"Ford","Mustang",1998);
–document.write("Your car is a " + myCar.year + " " + myCar.make + " " + myCar.model + ".");
–-Method apply (Member function)
§function TopClass (name, value) {
§ this.name = name;
§ this.value = value;
§ this.getAlertData = function () {
§ alert(this.name);
§ alert(this.value);
§ }
§}
§function SubClass (name, value, dept) {
§ this.dept = dept;
§ TopClass.apply(this, arguments);
§ this.getView = function () {
§ this.getAlertData();
§ }
§}
§//SubClass.prototype = new TopClass();
§var objSubClass = new SubClass("상위클래스","super","community");
§objSubClass.getView();
–-Method call
§Function.call(thisArg[, arg1[, arg2[, ...]]])
–function car(make, model, year) {
– this.make = make, this.model = model, this.year = year;
–}
–
–function hireCar(carNo, make, model, year) {
– this.carNo = carNo, car.call(this, make, model, year);
–}
•-Prototype
–Profiler = function () {..}
–Profiler.prototype.name = function () {..}
•-Namespace
–YAHOO = {};
•-new operator
–function object(o) {
– function F() {};
– F.prototype = o;
– return new F();
–}
–classA = new Profiler(); or classA = object(Profiler);
-Encapsulate (Parasitic)
YAHOO = {};
YAHOO.Trivia = function () {
var privateVar = "showPoser";
function privateNextPoser() {
alert("getNextPoser");
}
return {
getNextPoser: function (cat, diff) {
privateNextPoser();
},
showPoser: function () {
alert(privateVar);
}
};
} ();
function getFunc() {
var oObj = YAHOO.Trivia;
oObj.getNextPoser();
} |
YAHOO = {};
YAHOO.Trivia = function () {
var privateVar = "showPoser";
function privateNextPoser() {
alert("getNextPoser");
}
return {
getNextPoser: function (cat, diff) {
privateNextPoser();
},
showPoser: function () {
alert(privateVar);
}
};
};
function getFunc() {
var oObj = new YAHOO.Trivia();
oObj.getNextPoser();
} |
-Singletons
var singleton = function () {
var privateVariable;
function privateFunction(x) {
...privateVariable...
}
return {
firstMethod: function (a, b) {
...privateVariable...
},
secondMethod: function (c) {
...privateFunction()...
}
};
}();
ITWeb/개발일반 2008. 2. 20. 16:47
•자바스크립트 내장 객체
–-Number
–-String
–-Date
–-Array
–-Boolean
–-Math
–-RegExp
•브라우저 객체 모델(BOM) 객체
-window
§document
–forms
–cookie
–links/anchors
–images
§navigator
§location
§frames
§screen
§history
The Document Object Model (DOM) is an API for HTML and XML documents.
-Core DOM
§defines a standard set of objects for any structured document
-XML DOM
§defines a standard set of objects for XML documents
-HTML DOM
§defines a standard set of objects for HTML documents
•DOM 객체
–DOM Level 1,2,3
§DOM1 : 1997~1998
§DOM2 : 2000~2003
§DOM3 : 2004
Specification |
|
DOM Level 1 |
DOM Level 1 (SE) |
|
DOM Level 2 Core |
DOM Level 2 HTML |
DOM Level 2 Views |
DOM Level 2 Style |
DOM Level 2 Events |
DOM Level 2 Traversal-Range |
|
DOM Level 3 Requirements |
DOM Level 3 Core |
DOM Level 3 Events |
DOM Level 3 Load and Save |
DOM Level 3 Validation |
DOM Level 3 XPath |
DOM Level 3 Views |
•개발자가 만든 사용자 정의 객체
–-JavaScript objects 와 prototype.
§YAHOO.util.Connect = {
– _msxml_progid:[],
– _http_headers:{},
– _has_http_headers:false,
– setProgId:function(){…}
–}
§Profiler = function () {
– var getProfilers; // private
– this.getProfiler = function () {…} // public
–}
§String.prototype.trim = functoin () {…}
§Profiler = new Object();
–Profiler.title = “YAHOO”;
–Profiler.author = “Jerry”;
ITWeb/개발일반 2008. 2. 20. 16:36
Objects
–-Everything else is objects
–-Objects can contain data and methods
–-Objects can inherit from other objects.
•Collections
–-An object is an unordered collection of name/value pairs
–-Names are strings
–-Values are any type, including other objects
–-Good for representing records and trees
–-Every object is a little database
§var FUNC = function () {
§ var objDiv = document.createElement("div");
§ this.getCollection = function ( collection ) {
§ for ( i in collection ) {
§ objDiv.innerHTML += "name : " + i + "<br>value : " + collection[i] + "<br>";
§ }
§ document.body.appendChild(objDiv);
§ }
§}
§FUNC = new FUNC();
•Object Literals
–-Object literals are wrapped in { }
–-Names can be names or strings
–-Values can be expressions
–-: separates names and values
–-, separates pairs
–-Object literals can be used anywhere a value can appear
•Linkage
–-Objects can be created with a secret link to another object.
–-If an attempt to access a name fails, the secret linked object will be used.
–-The secret link is not used when storing. New members are only added to the primary object.
–-The object(o) function makes a new empty object with a link to object o.
function object(o) {
function F() {};
F.prototype = o;
return new F();
}
•JavaScript Invocations
–-If a function is called with too many arguments, the extra arguments are ignored.
–-If a function is called with too few arguments, the missing values will be undefined.
–-There is no implicit type checking on the arguments.
–-There are four ways to call a function:
§Function form
–functionObject(arguments)
§Method form
–thisObject.methodName(arguments)
–thisObject["methodName"](arguments)
§Constructor form
–new functionObject(arguments)
§Apply form
–functionObject.apply(thisObject, [arguments])
•JavaScript this
–-this is an extra parameter. Its value depends on the calling form.
–-this gives methods access to their objects.
–-this is bound at invocation time.
Invocation form |
this |
function |
the global object |
method |
the object |
constructor |
the new object |
JavaScript typeof
type |
typeof |
object |
'object' |
function |
'function' |
array |
'object' |
number |
'number' |
string |
'string' |
boolean |
'boolean' |
null |
'object' |
undefined |
'undefined' |
•Closure
–-The scope that an inner function enjoys continues even after the parent functions have returned.
–-This is called closure.
§function getClosure () {
§ var num = 1;
§ var getAlert = function() { num++; alert(num); }
§ num++;
§ return getAlert();
§}
§<input type="button" value="getClosure" onclick="getClosure();">
–function fade(id) {
– var dom = document.getElementById(id),
– level = 1;
– function step () {
– var h = level.toString(16);
– dom.style.backgroundColor =
– '#FFFF' + h + h;
– if (level < 15) {
– level += 1;
– setTimeout(step, 100);
– }
– }
– setTimeout(step, 100);
–}
•JavaScript Namespace
–-Every object is a separate namespace.
–-Use an object to organize your variables and functions.
§var YAHOO = {};
§YAHOO.url = "http://www.yahoo.com";
§YAHOO.getUrl = function () {
§ alert(this.url);
§ return this.url;
§}
§<input type="button" value="YAHOO.getUrl();" onclick="YAHOO.getUrl();">
•Inheritance
–-Linkage provides simple inheritance.
–-Prototypal inheritance.
–-Parasitic inheritance.
–-Method apply(), call().
ITWeb/개발일반 2008. 2. 20. 16:30
•Events
•Try…Catch
–try {
–} catch (err) {
–// err.name, err.message
–// err.number, err.description
–}
•Throw
–try {
– throw "error1";
–} catch (err) {
– if ( err == "error1" ) {
– alert("error1 발생");
– }
–}
JavaScript Special Text
Code |
Outputs |
\' |
single quote |
\" |
double quote |
\& |
ampersand |
\\ |
backslash |
\n |
new line |
\r |
carriage return |
\t |
tab |
\b |
backspace |
\f |
form feed |
ITWeb/개발일반 2008. 2. 20. 16:28
•JavaScript Loops
–for
§for (var=startvalue;var<=endvalue;var=var+increment) {
§ code to be executed
§}
§for (variable in object) {
§ code to be executed
§}
–while
§while (var<=endvalue) {
§ code to be executed
§}
§do {
§ code to be executed
§} while (var<=endvalue);
–break / continue (break label / continue label)
§for (i=0;i<=10;i++) {
§ if (i==3) {
§ break;
§ }
§ document.write("The number is " + I + "<br />");
§}
§forLable : for (i=0;i<=10;i++) {
§ if (i==3) {
§ continue forLable;
§ }
§ document.write("The number is " + I + "<br />");
§}
–
–with
§with ( document ) { // 반복 시킬 객체
§ write(“생략된 객체 반복1<br>”); // 구문
§ write(“생략된 객체 반복2<br>”); // 구문
§}
ITWeb/개발일반 2008. 2. 20. 16:22
•JavaScript Functions
–-Functions are first-class objects
–-Functions can be passed, returned, and stored just like any other value
–-Functions inherit from Object and can store name/value pairs.
–-A function is a reusable code-block that will be executed by an event, or when the function is called.
§function functionname(var1,var2,...,varX) {
§ some code
§ or
§ some code
§ return RETURNVALUE;
§}
–-function foo() {} expands to var foo = function foo() {}
•Inner Functions
-Functions do not all have to be defined at the top level (or left edge).
-Functions can be defined inside of other functions.
-Scope
§ An inner function has access to the variables and parameters of functions that it is contained within.
§ This is known as Static Scoping or Lexical Scoping.
ITWeb/개발일반 2008. 2. 20. 16:12
•JavaScript If…Else Statements
–if ( CONDITION ) {
–}
–if ( CONDITION ) {
–} else {
–}
–if ( CONDITION ) {
–} else if ( CONDITION ) {
–}
–if ( CONDITION ) {
–} else if ( CONDITION ) {
–} else {
–}
•JavaScript Switch Statement
–switch(n) {
– case 1:
– execute code block 1
– break;
– case 2:
– execute code block 2
– break;
– default:
– code to be executed if n is
– different from case 1 and 2
–}
JavaScript Operators
Operator |
Description |
Example |
Result |
+ |
Addition |
x=2 y=2 x+y |
4 |
- |
Subtraction |
x=5 y=2 x-y |
3 |
* |
Multiplication |
x=5 y=4 x*y |
20 |
/ |
Division |
15/5 5/2 |
3 2.5 |
% |
Modulus (division remainder) |
5%2 10%8 10%2 |
1 2 0 |
++ |
Increment |
x=5 x++ |
x=6 |
-- |
Decrement |
x=5 x-- |
x=4 |
Operator |
Example |
Is The Same As |
= |
x=y |
x=y |
+= |
x+=y |
x=x+y |
-= |
x-=y |
x=x-y |
*= |
x*=y |
x=x*y |
/= |
x/=y |
x=x/y |
%= |
x%=y |
x=x%y |
Operator |
Description |
Example |
== |
is equal to |
5==8 returns false |
=== |
is equal to (checks for both value and type) |
x=5 y="5" x==y returns true x===y returns false |
!= |
is not equal |
5!=8 returns true |
> |
is greater than |
5>8 returns false |
< |
is less than |
5<8 returns true |
>= |
is greater than or equal to |
5>=8 returns false |
<= |
is less than or equal to |
5<=8 returns true |
–-&&
§ If first operand is truthy
– then result is second operand
– else result is first operand
–-||
§ If first operand is truthy
– then result is first operand
– else result is second operand
Operator |
Description |
Example |
&& |
and |
x=6 y=3 (x < 10 && y > 1) returns true |
|| |
or |
x=6 y=3 (x==5 || y==5) returns false |
! |
not |
x=6 y=3
!(x==y) returns true |
-String
§txt1="What a very";
§txt2="nice day!";
§txt3=txt1+txt2;
-Conditional
§variablename = (condition) ? value1 : value2;
-alert
§var result = alert("sometext");
§alert(result); // undefined
-confirm
§var result = confirm("sometext");
§alert(result); // 확인:true, 취소:false
-prompt
§var result = prompt("sometext", "defaultvalue");
§alert(result); // 확인:string, 취소:null
ITWeb/개발일반 2008. 2. 20. 15:57
•JavaScript Blocks
–{…}
–-JavaScript does not have block scope.
–-Blocks should only be used with structured statements
§function, if, switch, while, for, do, try
•JavaScript Comments
–-Single-Line comments : //
–-Multi-Line comments : /*…*/
•JavaScript Variables
–-Variables are "containers" for storing information.
–-var keyword is defined local variable.
ITWeb/개발일반 2008. 2. 20. 15:54
•JavaScript Where To
–-Scripts in the head section: Scripts to be executed when they are called, or when an event is triggered, go in the head section. When you place a script in the head section, you will ensure that the script is loaded before anyone uses it.
–-Scripts in the body section: Scripts to be executed when the page loads go in the body section. When you place a script in the body section it generates the content of the page.
–-Scripts in both the body and the head section: You can place an unlimited number of scripts in your document, so you can have scripts in both the body and the head section.
|